More Than Just Log
15 July 2022
●
2 min read
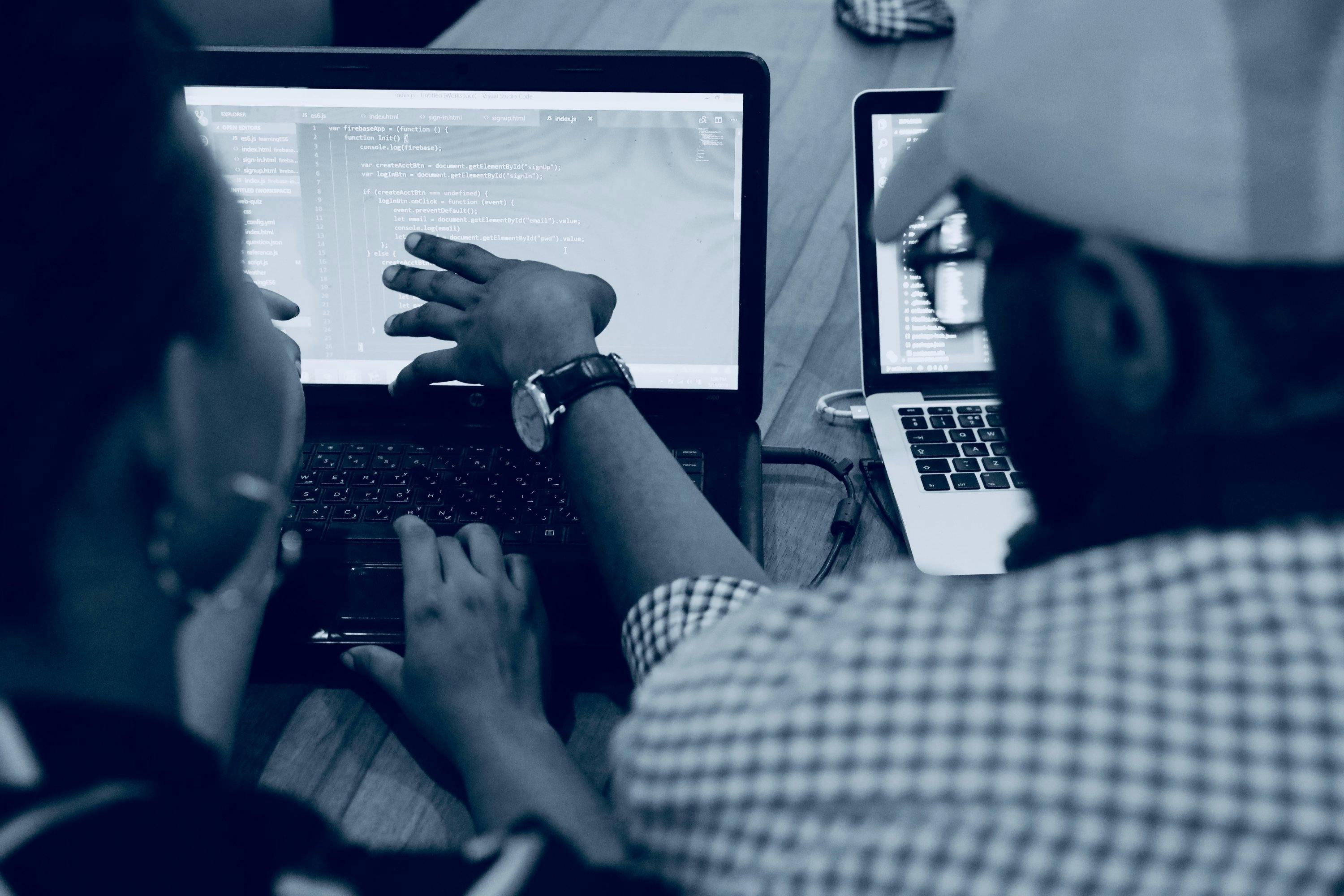
.table( )
console.table( ) allows you to print out objects and arrays in tabular form which then gives you the ability to visualise these in a better format than console.log( ).
const cities = [ { name: 'New York', population: 8177020 }, { name: 'London', population: 9540576 }, { name: 'Sydney', population: 5056571 }, ]; console.table(cities);
Passing an array of objects above produces a neat and tidy table. Columns represent the index and object keys and each row is populated with the values of the object.
.trace( )
Replace .log( ) with .trace( ) to debug errors in your code.
function app() { function doSomething() { let a = 1; let b = 2; addFunction(a, b); } function addFunction(a, b) { console.trace('In the add function'); return a + b; } doSomething(); } app();
The code above doesn't do much but with trace we can see the the copy of the call stack called the stack trace. With the stack trace we can visualise in which order the functions were called or invoked from the bottom to the top including the files from which they are called.
At the bottom of the stack we have an anonymous JavaScript function which is just the global namespace. Next the function app is called and put in the stack then the doSomething function and lastly the addFunction function.
Essentially, console.trace( ) will display the route taken to reach the point at which you use console.trace( ).